PHPMailer is an open-source library for sending emails in PHP applications. It is one of the best alternative to PHP’s native mail()
function. PHPMailer is used in CMS platforms like WordPress, Joomla, and Drupal. Other than PHMailer, you can also use Amazon SDK for PHP with SES to deliver emails.
How PHPMailer Works with WordPress
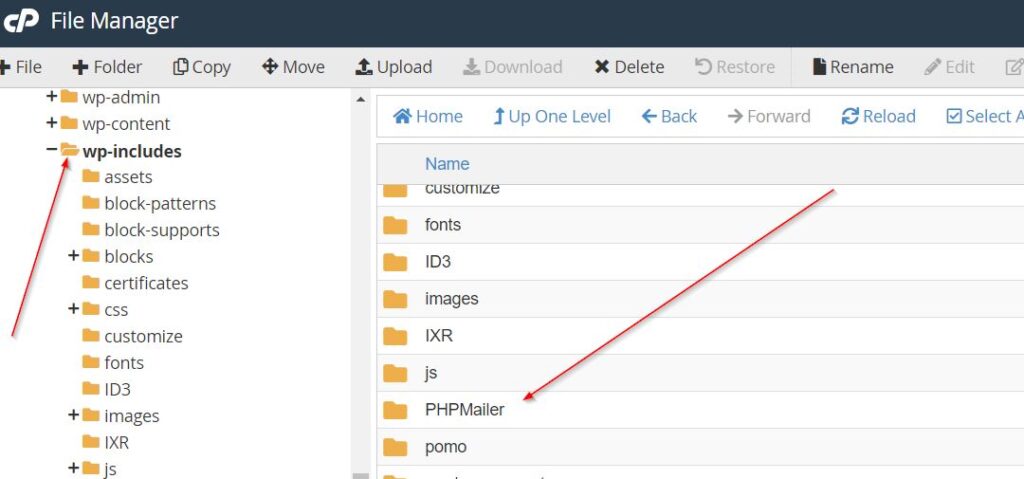
WordPress comes with a pre-integrated PHPMailer class located in the wp-includes
directory of your WordPress installation (wordpressfolder/wp-includes/class-phpmailer.php). This means you can use PHPMailer directly without additional installation steps. By default, WordPress uses your hosting provider’s SMTP server to send emails. However, relying on a shared server for sending large email volumes may lead to service suspensions due to email limits.
To enhance deliverability and avoid such issues, you can integrate PHPMailer with third-party SMTP services like Gmail, SendGrid, Elastic Mail, or Amazon SES.
Configuring PHPMailer in WordPress
Option 1: Using the functions.php File
You can initialize PHPMailer using the phpmailer_init
action hook. However, storing sensitive smtp credentials in the theme’s functions.php
file is risky, as anyone with access to your theme files could exploit these credentials, consider using the wp-config.php
option.
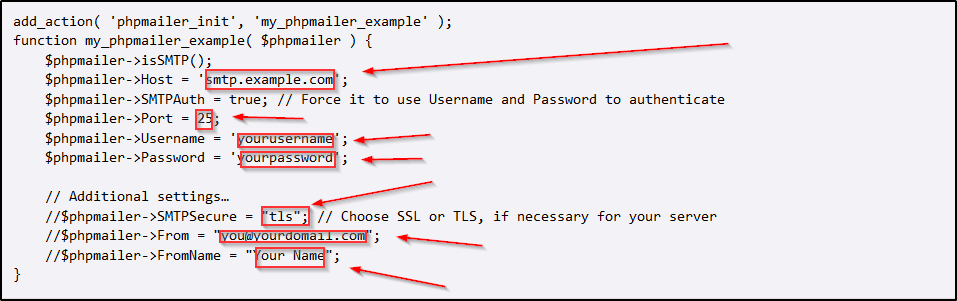
Sample Code to be added to functions.php
add_action('phpmailer_init', 'my_phpmailer_example');
'email@domain.com'
function my_phpmailer_example($phpmailer) {
$phpmailer->isSMTP();
$phpmailer->Host = 'smtp.domain.com';
$phpmailer->SMTPAuth = true;
$phpmailer->Port = '587';
$phpmailer->Username = 'smtp_username';
$phpmailer->Password = 'smtp_password';
$phpmailer->SMTPSecure = 'tls';
$phpmailer->From =;
'
$phpmailer->FromName =sender name
';
}
Replace the constants highlighted in red in the screenshot above, with your SMTP provider values. For better security, consider the wp-config.php
approach detailed below.
Option 2: Using wp-config.php
Using wp-confi
g.php
file is more secure for storing SMTP credentials as it resides outside the publicly accessible directories. Add the following code to your wp-config.php file:
define('SMTP_USER', 'user@example.com'); // SMTP username
define('SMTP_PASS', 'your-password'); // SMTP password
define('SMTP_HOST', 'smtp.example.com'); // SMTP server
define('SMTP_PORT', 587); // SMTP port (e.g., 25, 465, 587)
define('SMTP_SECURE', 'tls'); // Encryption type (tls or ssl)
define('SMTP_AUTH', true); // Enable SMTP authentication
define('SMTP_FROM', 'no-reply@example.com'); // Sender email address
define('SMTP_NAME', 'Example Name'); // Sender name
Features of PHPMailer
- Supports
To
,Cc
, andBcc
fields. - Attach files directly to emails.
- Allows sending formatted emails using HTML.
- UTF-8 Support
Setting Up PHPMailer in Control Panel
For non-WordPress projects (using manual installation not composer), follow the steps below. :
- Download PHPMailer latest version from PHPMailer’s GitHub page.
- Set up an email account in cPanel or use an email provider like Gmail.
- Create folder in public_html, for example, “mymailer” and place the extracted PHPMailer in the folder.
- Create a mail.php script for sending emails and place it in the same folder as PHMailer. Add the code below to the mail.php file and open your browser to test whether email sent.
require 'path/to/PHPMailer/src/PHPMailer.php';
require 'path/to/PHPMailer/src/SMTP.php';
require 'path/to/PHPMailer/src/Exception.php';
$mail = new PHPMailer\PHPMailer\PHPMailer();
try {
$mail->isSMTP();
$mail->Host = 'smtp.domain.com';
$mail->SMTPAuth = true;
$mail->Username = 'smtp-username';
$mail->Password = 'smt-your-password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
$mail->setFrom('name@domain.com', 'Your Name');
$mail->addAddress('customer@domain.com', 'Recipient Name');
$mail->Subject = 'PHMailer Test Email';
$mail->Body = 'I am testing whether PHPMailer works.';
$mail->send();
echo 'PHPMailer Message sent successfully.';
} catch (Exception $e) {
echo 'PHPMailer Message could not be delivered. Mailer Error: ' . $mail->ErrorInfo;
}
NOTE: If you are using Windows or installed PHPMailer using composer, then change the require path and $mail variable values to; require 'path/to/mymailer/PHPMailer/PHPMailerAutoload.php';
$mail = new PHPMailer(true);
Additional Email Deliveravility Notes
Makesure that you have added SPF and DKIM records to your DNS records for better email deliverability.
You can also make your work easier by using free self-hosted script and PHP application listed on email service providers like under open-source filter option.